To determine how much memory your app is currently using, look at the Persistent Bytes column for the category All Heap Allocations. The text is a little small in the screenshot, but it says the app is using 9.64 MB of memory, which is good for an iOS or Mac app. The Allocations instrument does not record OpenGL/ES or Metal texture memory. Total Virtual Memory. This one is tricky on Mac OS X because it doesn't use a preset swap partition or file like Linux. Here's an entry from Apple's documentation: Note: Unlike most Unix-based operating systems, Mac OS X does not use a preallocated swap partition for virtual memory. Instead, it uses all of the available space on the machine's.
Memory (RAM) and storage (hard disk / SSD) are not related to one another.
That Mac has plenty of available storage. If you are running low on memory Activity Monitor can be used to identify the memory-intensive processes causing that warning.
To learn how to use Activity Monitor please read the Activity Monitor User Guide. For memory usage, refer to View memory usage in Activity Monitor on Mac.
Once you determine the memory-intensive process or processes, a solution can be provided. WIthout that information it is premature to draw any conclusions, but the number one explanation for that warning is having inadvertently installed adware. To learn how to recognize adware so that you do not install it, please read How to install adware - Apple Community.
Jan 1, 2019 7:49 AM
I found myself needing to measure the memory usage of a programthroughout its run time and was surprised that I didn't find a tool outthere already that did what I wanted. After a bunch of work, I figured out that it's easy to roll ityourself. In the hope of saving somebody that work in the future, here'show to do it. This post describes how to run a process, sample its memory throughoutits run using only bash and unix tools, and plot the results usinggnuplot
.
The process we'll measure
In my case, I was measuring an ETL process, but this technique isequally applicable to any process on your system. For demonstration purposes, we need a meaningless process that takes ashort while. The process we'll measure is:
grep -ris 'banana' /usr/bin

On my system, this process takes about four seconds and returns no results.This grep search has three flags:
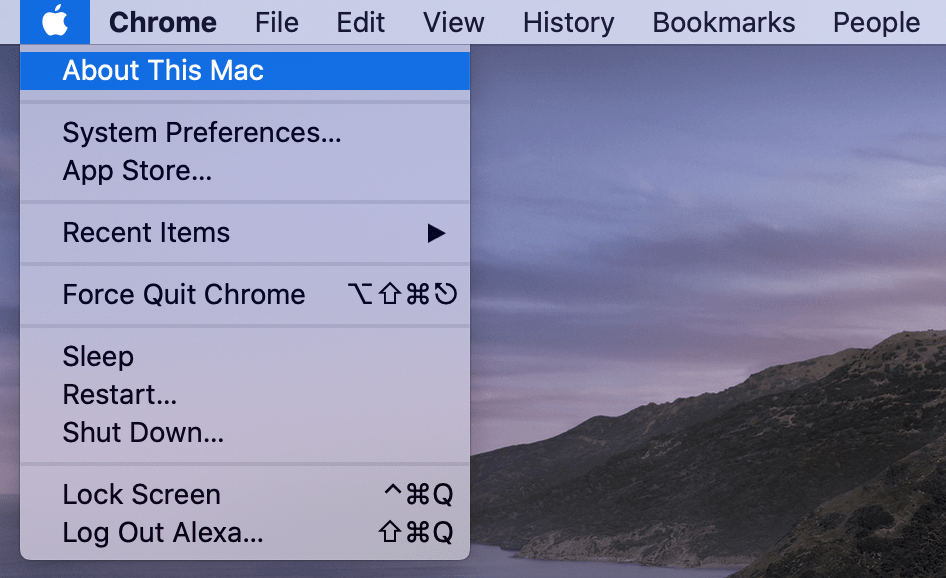
-r
: search recursively. This means that grep won't stop atjust searching the files in /usr/bin, but will search all subdirectories.-i
: search case insensitively. I did this so the process wouldrun a bit longer.-s
: silent mode. This means nonexistent and unreadable files areignored.
What is memory even? (A refresher)
On a modern OS, each process gets a virtual addressspace. This means it hasaccess to a vast array of memory, which may or may not actually be stored onthe RAM of the physicalcomputer. The OS will, at its discretion, move memorypages fromyour process' virtual memory into and out of physical RAM. One app may share memory with another; if two programs both load thesame shared library, the OS will (probably, at its discretion) load onlyone copy into RAM.
There are two principal measurements1 of your program'ssize, using this information:
Virtual size: The total virtual address space allocated to yourprogram
Resident set size: The total memory space currently resident in RAM andassociated with your program
The measurement we want is rss
: the resident set size of the programwe're studying.
ps
There are many tools to investigate a running program's memory, but let'sexamine ps
. Its main virtues are that it's simple and it'savailable everywhere, in reasonably cross-platform fashion.
We can list the processes our user owns, sorted by memory: ps -m
That's handy, but it doesn't actually show memory usage, so let's tell it toshow some columns: ps -m -o pid,vsz,rss,%mem,command
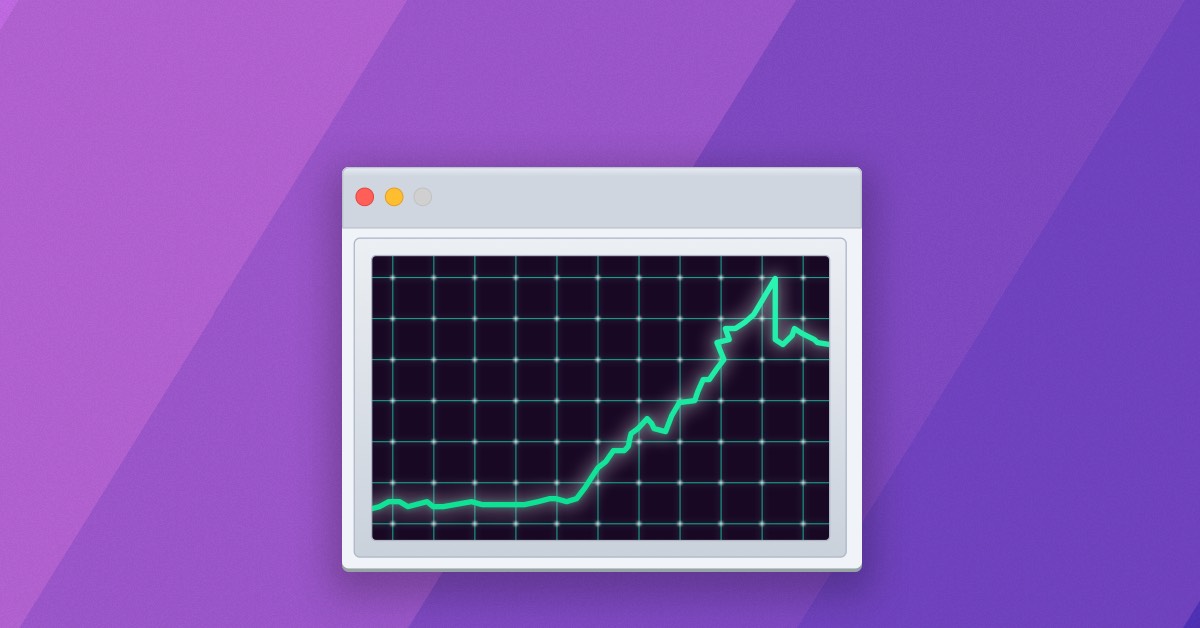
In this case, we've asked it to show us pid
, the process id ofeach displayed process; vsz
, its virtual address size;rss
, its resident set size; %mem
, the percent ofphysical memory occupied by that process' resident set; andcommand
, the full command that is running. The -o
option to ps allows us to specify what columns we want todisplay; look at the man page to see the full list of what's available. The final piece we need is to limit this list by process id with the-p
flag.
Here's a command to get the pid of the top memory-using process on our system:ps -o pid= | head -n1
2
Finally, we can use that to construct a command to print out the rss
of theprocess we own that's using the most memory: ps -opid,vsz,rss,%mem,command -p $(ps -m -o pid= | head-n1)
3
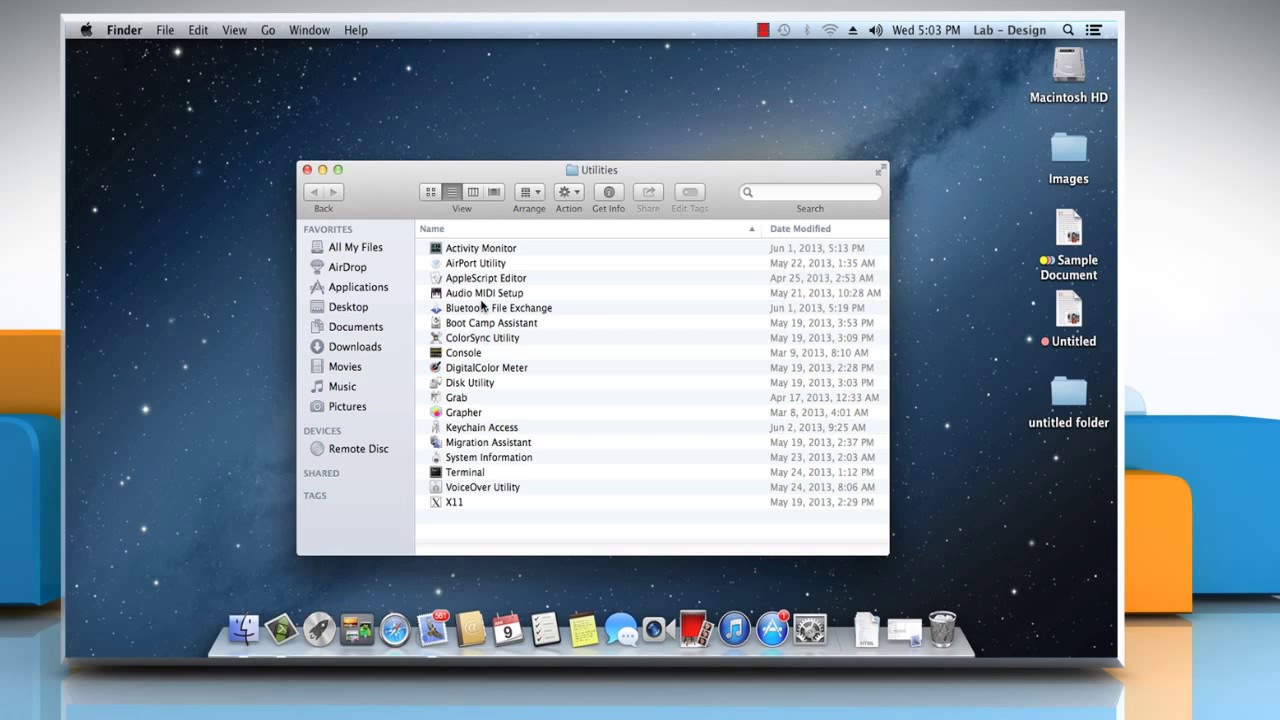
Sampling
Now that we know how to measure a given process' rss
, we can go back toour initial idea of measuring the memory usage of grep -ris 'banana' /usr/bin
. Let's start writing a bash script:
This script does the following:
Tells the OS to use bash to run the script
Tells bash to quit if any command fails (
set -e
)Runs the grep command we have previously talked about and puts it into thebackground (
&
)Gets the process identifier (PID) of the most recent command put into the background(
$!
) and saves it to the variablepid
Prints the grep's memory usage using the PID we stored
That's a great start!
To measure memory usage over time, we need to run that in a loop, limit theoutput a bit, and append to a file which stores the measured values. We'll need a couple of tools for this:
date +%s
: outputs the time in seconds since the epochprintf
: bash's super-handyprintf
functionmktemp
: a unix tool that we'll use to make a temporary file forour memory trace log
Here's a script that starts the process, creates a log file, samples the memorysize every tenth of a second, and saves it to the log:
Memory Usage Widget
Graphing
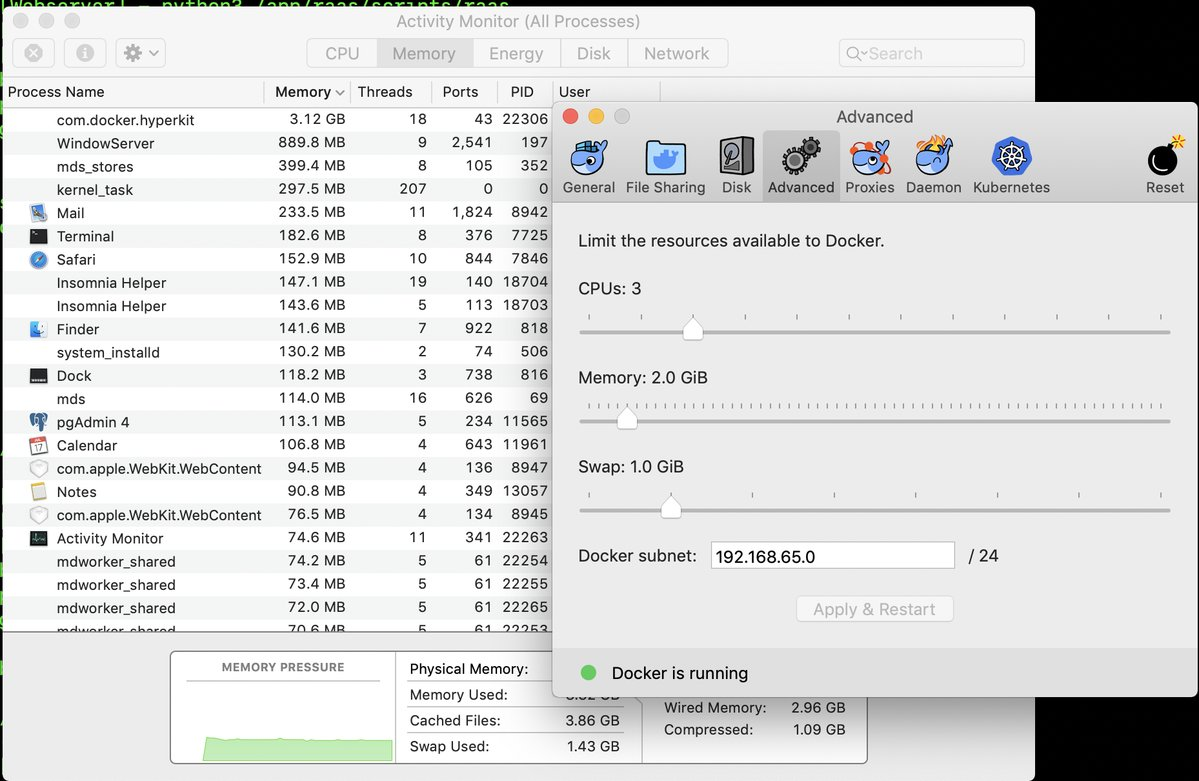
On my system, this process takes about four seconds and returns no results.This grep search has three flags:
-r
: search recursively. This means that grep won't stop atjust searching the files in /usr/bin, but will search all subdirectories.-i
: search case insensitively. I did this so the process wouldrun a bit longer.-s
: silent mode. This means nonexistent and unreadable files areignored.
What is memory even? (A refresher)
On a modern OS, each process gets a virtual addressspace. This means it hasaccess to a vast array of memory, which may or may not actually be stored onthe RAM of the physicalcomputer. The OS will, at its discretion, move memorypages fromyour process' virtual memory into and out of physical RAM. One app may share memory with another; if two programs both load thesame shared library, the OS will (probably, at its discretion) load onlyone copy into RAM.
There are two principal measurements1 of your program'ssize, using this information:
Virtual size: The total virtual address space allocated to yourprogram
Resident set size: The total memory space currently resident in RAM andassociated with your program
The measurement we want is rss
: the resident set size of the programwe're studying.
ps
There are many tools to investigate a running program's memory, but let'sexamine ps
. Its main virtues are that it's simple and it'savailable everywhere, in reasonably cross-platform fashion.
We can list the processes our user owns, sorted by memory: ps -m
That's handy, but it doesn't actually show memory usage, so let's tell it toshow some columns: ps -m -o pid,vsz,rss,%mem,command
In this case, we've asked it to show us pid
, the process id ofeach displayed process; vsz
, its virtual address size;rss
, its resident set size; %mem
, the percent ofphysical memory occupied by that process' resident set; andcommand
, the full command that is running. The -o
option to ps allows us to specify what columns we want todisplay; look at the man page to see the full list of what's available. The final piece we need is to limit this list by process id with the-p
flag.
Here's a command to get the pid of the top memory-using process on our system:ps -o pid= | head -n1
2
Finally, we can use that to construct a command to print out the rss
of theprocess we own that's using the most memory: ps -opid,vsz,rss,%mem,command -p $(ps -m -o pid= | head-n1)
3
Sampling
Now that we know how to measure a given process' rss
, we can go back toour initial idea of measuring the memory usage of grep -ris 'banana' /usr/bin
. Let's start writing a bash script:
This script does the following:
Tells the OS to use bash to run the script
Tells bash to quit if any command fails (
set -e
)Runs the grep command we have previously talked about and puts it into thebackground (
&
)Gets the process identifier (PID) of the most recent command put into the background(
$!
) and saves it to the variablepid
Prints the grep's memory usage using the PID we stored
That's a great start!
To measure memory usage over time, we need to run that in a loop, limit theoutput a bit, and append to a file which stores the measured values. We'll need a couple of tools for this:
date +%s
: outputs the time in seconds since the epochprintf
: bash's super-handyprintf
functionmktemp
: a unix tool that we'll use to make a temporary file forour memory trace log
Here's a script that starts the process, creates a log file, samples the memorysize every tenth of a second, and saves it to the log:
Memory Usage Widget
Graphing
Now we have a logfile that contains two columns: seconds since programstart and memory usage in kB. To graph it, we'll use the handy toolgnuplot. It may not be the prettiest, butit's available everywhere and simple to use. The simplest gnuplot invocation that shows us a graph is: gnuplot -p -e'plot '$logfile' with lines'
-p:
leave the graph showing after gnuplot exits-e:
run this plotting script; here we tell it to make a line graph from our logfile, and it complies
We can also pass gnuplot a longer script on stdin
; here we use it to show a graph in our console by adding this command to the bottom of our script:
Here we can clearly see that grep's memory usage starts at about 2kB andjumps to about 1MB at the 2 second mark. Not bad for a short script!
Software To Measure Memory Usage Mac Pro
We can also output a fancier graph. Here's a gnuplot script that willoutput a PNG graph:
Customize the graph to your heart's content using the gnuplotdocumentation.
Conclusion
That's a brief trip into how we can use bash
andgnuplot
to trace and show a graph of a process' memory usagethroughout its lifetime. I hope you learned a trick or two.
Footnotes
1: On Linux there is a measurement called Proportional Set Size
which attempts to factor out the shared space used by your program. Ifprograms A and B have private memory of 500MB and share a 200MB library, thePSS will be 600MB for each. Mac does not really have this measurement, thoughyou can mostly figure it out usingvmmap
2: The equals following the output specifier tells ps
not toprint the header. From the BSD man page:
Keywords may be appended with an equals (=
) sign and a string. This causesthe printed header to use the specified string instead of the standardheader. If all keywords have empty header texts, no header line is written.
Check Mac Memory Usage
3: Yes, we could sort by memory and head
the output, butwe're going to need to specify a process ID later.
Further Explore These Topics
Older
The power of being naive
April 23, 2019
Software To Measure Memory Usage Mac Mini
Subject matter naiveté is our expertise. We're hired to be experts in process, creation, and problem solving, and the primary tool we use to do this work is asking questions.